Introduction:
Interviewer has ask about
method overriding related tricky question in many interview. Definition is
simple but many people get confused during interview.
I gets confused every time, even
though I have read many times. So I thought of writing this article to clear
all the doubts.
What is method overriding?
There are two type of polymorphism.
Method overloading and Method overriding. Method overriding is important
concept in C#. Method having same signature and inherited
from base class to derived classes is called method overriding.
Here same signature means method
name, number of arguments and type of arguments should be same.
Inheritance is required to achieve
the method overriding.
How to achieve?
Virtual and override keyword is used
for method overriding.
Override keyword is used with
virtual and abstract method, property and event.
Inheritance:
In simple term we can say
inheriting from parent class to derive class is called inheritance.
Three classes are mention here.
Base, Derived and Subderived class. Base class has Show method. Derive class
inherit base and subderive class inherit Derive class.
So Show() method is available for
all classes.
Example:
Code for above diagram is mention
here.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace
MethodOverriding
{
{
class Base
{
{
public void Show()
{
Console.WriteLine("Base");
{
Console.WriteLine("Base");
}
}
class
Derived : Base
{
{
}
class
SubDerived : Derived
{
{
}
class Program
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
class Program
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
b = new Derived();
b.Show();//Drived
b.Show();//Drived
SubDerived d = new SubDerived();
d.Show();//SubDerive
d.Show();//SubDerive
Console.ReadLine();
}
}
}
}
Output: Show() method is available
for both derive and subderive class.
Output will be Base, Base, Base as
shown below.
Hiding and Overriding Methods.
What will be output of this program.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace
MethodOverriding
{
{
class Base
{
public void Show()
{
Console.WriteLine("Base");
{
Console.WriteLine("Base");
}
}
class
Derived : Base
{
public void Show()
{
Console.WriteLine("Derived");
{
public void Show()
{
Console.WriteLine("Derived");
}
}
class
SubDerived : Derived
{
public void Show()
{
Console.WriteLine("SubDerived");
{
public void Show()
{
Console.WriteLine("SubDerived");
}
}
}
class
Program
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
Derived d = new Derived();
d.Show();//Drived
d.Show();//Drived
SubDerived sd = new SubDerived();
sd.Show();//SubDerive
sd.Show();//SubDerive
Console.WriteLine("---------------------------------");
b = d;
b.Show();//Base
b.Show();//Base
b = sd;
b.Show();//Base
Console.ReadLine();
}
}
}
}
Output: It will show warning for
both Show method of Derived class and sub derived class as shown below.
But output will be as mention:
Method
hiding(using new keyword)
Derive class overrides Base class.
Show() method of Derive class hides the Show() method of Base class in above
example. That’s way it is showing warning.
We can use new keyword if we
want to hide Show() method of Base class in Derived class intentionally.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace
MethodOverriding
{
{
class Base
{
public void Show()
{
Console.WriteLine("Base");
{
Console.WriteLine("Base");
}
}
class
Derived : Base
{
public new void Show()
{
Console.WriteLine("Derived");
{
public new void Show()
{
Console.WriteLine("Derived");
}
}
class
SubDerived : Derived
{
public new void Show()
{
Console.WriteLine("SubDerived");
{
public new void Show()
{
Console.WriteLine("SubDerived");
}
}
}
class
Program
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
Derived d = new Derived();
d.Show();//Drived
d.Show();//Drived
SubDerived sd = new SubDerived();
sd.Show();//SubDerive
sd.Show();//SubDerive
Console.WriteLine("---------------------------------");
b = d;
b.Show();//Base
b.Show();//Base
b = sd;
b.Show();//Base
Console.ReadLine();
}
}
}
}
Output: Output will be same as above example without any warning.
Output: Output will be same as above example without any warning.
Runtime polymorphism:
Main purpose of method overriding is
to achieve runtime polymorphism. Virtual and override keyword is used for this.
Show() method of Base class is
declare as virtual method. Derive class has derived from Base class using
override keyword. SubDerived class has overridden Derive class.
Here Base class instance b is
consider as game changer. Create object of Base class like Base b=new Base().
Initialize derive class object d to b and SubDrive class object sd to b.
Then see the actual magic.
Base b = new Base()
b.Show();//”Base”
b= new Derive();
b.Show();//”Drive”
b = new SubDerived();
b.Show();//”SubDerived”
Here you can notice that, what ever
object is assign to Base class object, respective method of that class is
called.
Example:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace
MethodOverriding
{
{
class Base
{
public virtual void Show()
{
Console.WriteLine("Base");
{
Console.WriteLine("Base");
}
}
class
Derived : Base
{
public override void Show()
{
Console.WriteLine("Derived");
{
public override void Show()
{
Console.WriteLine("Derived");
}
}
class
SubDerived : Derived
{
public override void Show()
{
Console.WriteLine("SubDerived");
{
public override void Show()
{
Console.WriteLine("SubDerived");
}
}
}
class
Program
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
Derived d = new Derived();
d.Show();//Drived
d.Show();//Drived
SubDerived sd = new SubDerived();
sd.Show();//SubDerive
sd.Show();//SubDerive
Console.WriteLine("---------------------------------");
b = d;
b.Show();//Drived
b.Show();//Drived
b = sd;
b.Show();//SubDerive
Console.ReadLine();
}
}
}
}
Output:
Some Tricky Question:
1. What will be output of following
program.
class Base
{
{
public virtual void Show()
{
Console.WriteLine("Base");
{
Console.WriteLine("Base");
}
}
class
Derived : Base
{
public void Show()
{
Console.WriteLine("Derived");
{
public void Show()
{
Console.WriteLine("Derived");
}
}
class
SubDerived : Derived
{
public new void Show()
{
Console.WriteLine("SubDerived");
{
public new void Show()
{
Console.WriteLine("SubDerived");
}
}
}
class Program
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
Derived d = new Derived();
d.Show();//Drived
d.Show();//Drived
SubDerived sd = new SubDerived();
sd.Show();//SubDerive
sd.Show();//SubDerive
Console.WriteLine("---------------------------------");
b = d;
b.Show();//Drived
b.Show();//Drived
b = sd;
b.Show();//SubDerive
Console.ReadLine();
}
}
}
Output: There will be compilation
warning.
but output will be like this screen.
2. What will be output of following
program.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace
MethodOverridingAndHidingTrickyQuestion
{
class Base
{
{
class Base
{
public virtual void Show()
{
Console.WriteLine("Base");
{
Console.WriteLine("Base");
}
}
class
Derived : Base
{
public override void Show()
{
Console.WriteLine("Derived");
{
public override void Show()
{
Console.WriteLine("Derived");
}
}
class
SubDerived : Derived
{
public new void Show()
{
Console.WriteLine("SubDerived");
{
public new void Show()
{
Console.WriteLine("SubDerived");
}
}
}
class
Program
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
Derived d = new Derived();
d.Show();//Drived
d.Show();//Drived
SubDerived sd = new SubDerived();
sd.Show();//SubDerive
sd.Show();//SubDerive
Console.WriteLine("---------------------------------");
b = d;
b.Show();//Derived
b.Show();//Derived
b = sd;
b.Show();//Derived
Console.ReadLine();
}
}
}
}
}
Output: Output is strange. See the
output screen. This is left to readers to find the reason
behind this.
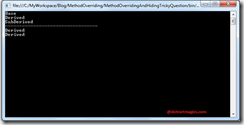
3. What will be output of below
program.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace
MethodOverridingAndHidingTrickyQuestion
{
class Base
{
{
class Base
{
public void Show()
{
Console.WriteLine("Base");
{
Console.WriteLine("Base");
}
}
class
Derived : Base
{
public new virtual void Show()
{
Console.WriteLine("Derived");
{
public new virtual void Show()
{
Console.WriteLine("Derived");
}
}
class
SubDerived : Derived
{
public override void Show()
{
Console.WriteLine("SubDerived");
{
public override void Show()
{
Console.WriteLine("SubDerived");
}
}
}
class
Program
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
{
static void Main(string[] args)
{
Base b = new Base();
b.Show(); // Base
Derived d = new Derived();
d.Show();//Drived
d.Show();//Drived
SubDerived sd = new SubDerived();
sd.Show();//SubDerive
sd.Show();//SubDerive
Console.WriteLine("---------------------------------");
b = d;
b.Show();//Base
b.Show();//Base
b = sd;
b.Show();//Base
Console.ReadLine();
}
}
}
}
}
Output: Here output is little
strange for these 2 lines.
b = d;
b.Show();//Base
b.Show();//Base
Base class doesn’t has virtual function. Derive class has implemented virtual
method.
derive class object d is assign to base class object b and method show() gets
called but same method with same signature is present in Base class also
so it
calls Show() method of base class.
b = sd;
b.Show();//Base
b.Show();//Base
Same happens for SubDrived
class also.